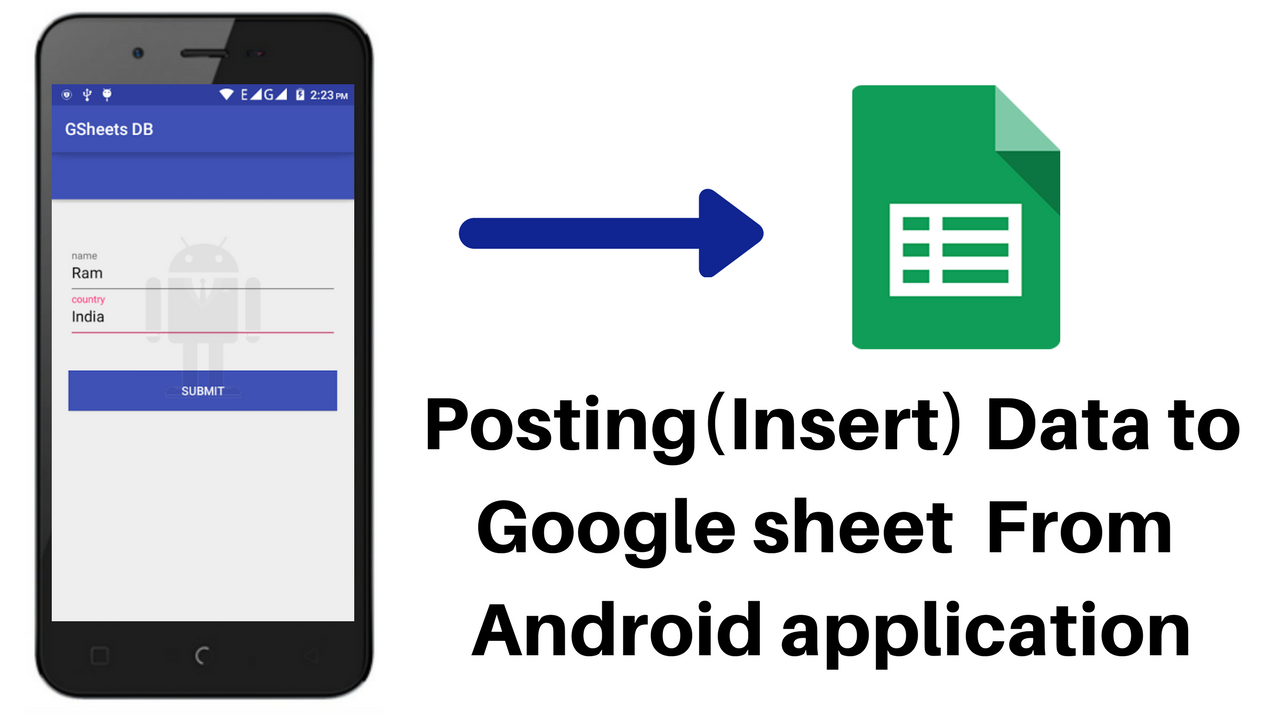
We have seen about fetching data from Google spread sheet in Part 1. Here I am going to explain about Posting data into Google Sheets from your android device.
Here It Consists of 2 Parts
- Creating app script (Google Script) that inserts data into Spread Sheet and publishing it as web app.
- Using the url send Http Post request and pass the parameter from Android App. [maxbutton id=”1″]
1. Creating App Script
Note : create your own script or you can use the script I have published.
Step 1 : Go to App script console and create new project in app script .
Step 2 : Insert the below Code which inserts data to Spread sheet.
function doGet(e){ return handleResponse(e); } //Recieve parameter and pass it to function to handle function doPost(e){ return handleResponse(e); } // here handle with parameter function handleResponse(request) { var output = ContentService.createTextOutput(); //create varibles to recieve respective parameters var name = request.parameter.name; var country = request.parameter.country; var id = request.parameter.id; //open your Spread sheet by passing id var ss= SpreadsheetApp.openById(id); var sheet=ss.getSheetByName("Sheet1"); //add new row with recieved parameter from client var rowData = sheet.appendRow([name,country]); var callback = request.parameters.callback; if (callback === undefined) { output.setContent(JSON.stringify("Success")); } else { output.setContent(callback + "(" + JSON.stringify("Success") + ")"); } output.setMimeType(ContentService.MimeType.JSON); return output; }
Step 3 : Now Publish (Deploy) it as web App with Access to anyone.
Step 4 : Copy the link and save it for further use in Step
you can use post man tool to verify doGet and doPost activities.
2. Android Part
Note : Since I am enhancing previous project. You can refer previous part before reading further.
and I have renamed MainActivity.java and main.xml files. Everything else remains same.
Step 1 : Create form.xml to take inputs from user.
<android.support.design.widget.CoordinatorLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:app="http://schemas.android.com/apk/res-auto" android:layout_width="match_parent" android:layout_height="match_parent"> <android.support.design.widget.AppBarLayout android:layout_width="match_parent" android:layout_height="wrap_content" android:theme="@style/ThemeOverlay.AppCompat.Dark.ActionBar"> <android.support.v7.widget.Toolbar android:id="@+id/toolbar" android:layout_width="match_parent" android:layout_height="?attr/actionBarSize" android:background="?attr/colorPrimary" app:layout_scrollFlags="scroll|enterAlways" app:popupTheme="@style/ThemeOverlay.AppCompat.Light" /> </android.support.design.widget.AppBarLayout> <LinearLayout android:layout_width="fill_parent" android:layout_height="match_parent" android:layout_marginTop="?attr/actionBarSize" android:orientation="vertical" android:paddingLeft="20dp" android:paddingRight="20dp" android:paddingTop="60dp"> <android.support.design.widget.TextInputLayout android:id="@+id/input_layout_name" android:layout_width="match_parent" android:layout_height="wrap_content"> <EditText android:id="@+id/input_name" android:layout_width="match_parent" android:layout_height="wrap_content" android:singleLine="true" android:hint="name" /> </android.support.design.widget.TextInputLayout> <android.support.design.widget.TextInputLayout android:id="@+id/input_layout_email" android:layout_width="match_parent" android:layout_height="wrap_content"> <EditText android:id="@+id/input_country" android:layout_width="match_parent" android:layout_height="wrap_content" android:hint="country" /> </android.support.design.widget.TextInputLayout> <Button android:id="@+id/btn_submit" android:layout_width="fill_parent" android:layout_height="wrap_content" android:text="Submit" android:background="@color/colorPrimary" android:layout_marginTop="40dp" android:textColor="@android:color/white"/> </LinearLayout> </android.support.design.widget.CoordinatorLayout>
Step 2 : Create PostData.java class to handle input and post it into server using deployed web app. Thats it with the requirement.
package androidlabs.gsheets2.Post; import android.app.ProgressDialog; import android.os.AsyncTask; import android.os.Bundle; import android.support.v7.app.AppCompatActivity; import android.util.Log; import android.view.View; import android.widget.Button; import android.widget.EditText; import android.widget.TextView; import android.widget.Toast; import org.json.JSONObject; import java.io.BufferedReader; import java.io.BufferedWriter; import java.io.InputStreamReader; import java.io.OutputStream; import java.io.OutputStreamWriter; import java.net.HttpURLConnection; import java.net.URL; import java.net.URLEncoder; import java.util.Iterator; import javax.net.ssl.HttpsURLConnection; import androidlabs.gsheets2.R; public class PostData extends AppCompatActivity { private ProgressDialog progress; TextView tvName; TextView tvCountry; Button button; String name; String country; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.form); button=(Button)findViewById(R.id.btn_submit); tvName=(EditText)findViewById(R.id.input_name); tvCountry=(EditText)findViewById(R.id.input_country); button.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View v) { name = tvName.getText().toString(); country=tvCountry.getText().toString(); new SendRequest().execute(); } } ); } public class SendRequest extends AsyncTask<String, Void, String> { protected void onPreExecute(){} protected String doInBackground(String... arg0) { try{ URL url = new URL("https://script.google.com/macros/s/AKfycbx-Nu9l0zuZ0olaCeuS5iBHoaLoDEXtQhvw6TmUokuQj_uR7Uw/exec"); // https://script.google.com/macros/s/AKfycbyuAu6jWNYMiWt9X5yp63-hypxQPlg5JS8NimN6GEGmdKZcIFh0/exec JSONObject postDataParams = new JSONObject(); //int i; //for(i=1;i<=70;i++) // String usn = Integer.toString(i); String id= "1hYZGyo5-iFpuwofenZ6s-tsaFPBQRSx9HQYydigA4Dg"; postDataParams.put("name",name); postDataParams.put("country",country); postDataParams.put("id",id); Log.e("params",postDataParams.toString()); HttpURLConnection conn = (HttpURLConnection) url.openConnection(); conn.setReadTimeout(15000 /* milliseconds */); conn.setConnectTimeout(15000 /* milliseconds */); conn.setRequestMethod("POST"); conn.setDoInput(true); conn.setDoOutput(true); OutputStream os = conn.getOutputStream(); BufferedWriter writer = new BufferedWriter( new OutputStreamWriter(os, "UTF-8")); writer.write(getPostDataString(postDataParams)); writer.flush(); writer.close(); os.close(); int responseCode=conn.getResponseCode(); if (responseCode == HttpsURLConnection.HTTP_OK) { BufferedReader in=new BufferedReader(new InputStreamReader(conn.getInputStream())); StringBuffer sb = new StringBuffer(""); String line=""; while((line = in.readLine()) != null) { sb.append(line); break; } in.close(); return sb.toString(); } else { return new String("false : "+responseCode); } } catch(Exception e){ return new String("Exception: " + e.getMessage()); } } @Override protected void onPostExecute(String result) { Toast.makeText(getApplicationContext(), result, Toast.LENGTH_LONG).show(); } } public String getPostDataString(JSONObject params) throws Exception { StringBuilder result = new StringBuilder(); boolean first = true; Iterator<String> itr = params.keys(); while(itr.hasNext()){ String key= itr.next(); Object value = params.get(key); if (first) first = false; else result.append("&"); result.append(URLEncoder.encode(key, "UTF-8")); result.append("="); result.append(URLEncoder.encode(value.toString(), "UTF-8")); } return result.toString(); } }
But to give UI to select Inserion and View I am adding one more xml and class
Step 3 : Create main_page.xml to add 2 buttons to choose.
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="match_parent" android:layout_height="match_parent" android:gravity="center" android:orientation="vertical" > <Button android:id="@+id/insertUser" android:layout_width="match_parent" android:layout_height="wrap_content" android:layout_gravity="center" android:layout_marginLeft="25dp" android:layout_marginRight="25dp" android:background="#37a8f7" android:text="View User" android:layout_marginTop="15dp" android:textColor="#fff" /> <Button android:id="@+id/viewUser" android:layout_width="match_parent" android:layout_height="wrap_content" android:layout_gravity="center" android:layout_marginLeft="25dp" android:layout_marginRight="25dp" android:background="#37a8f7" android:text="Add User" android:layout_marginTop="15dp" android:textColor="#fff" /> </LinearLayout>
Step 4 : Create MainPage.java to handle buttons. Use Intent objects to Navigate to respective activities.
package androidlabs.gsheets2; import android.content.Intent; import android.os.Bundle; import android.support.v7.app.AppCompatActivity; import android.view.View; import android.widget.Button; import androidlabs.gsheets2.Post.PostData; /** * Created by ADJ on 2/21/2017. */ public class MainPage extends AppCompatActivity{ Button getData; Button sendData; protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.main_page); getData=(Button)findViewById(R.id.insertUser); sendData=(Button)findViewById(R.id.viewUser); getData.setOnClickListener(new View.OnClickListener(){ @Override public void onClick(View v) { Intent intent = new Intent(getApplicationContext(), UserList.class); startActivity(intent); } }); sendData.setOnClickListener(new View.OnClickListener(){ @Override public void onClick(View v) { Intent intent = new Intent(getApplicationContext(), PostData.class); startActivity(intent); } }); }; }
Step 5 : Then Modify in manifest file as below by including newly added Classes and change Launching Activity.
<manifest xmlns:android="http://schemas.android.com/apk/res/android" package="androidlabs.gsheets2"> <uses-permission android:name="android.permission.INTERNET" /> <uses-permission android:name="android.permission.ACCESS_NETWORK_STATE" /> <uses-permission android:name="android.permission.ACCESS_WIFI_STATE" /> <application android:icon="@drawable/logo_icon" android:label="@string/app_name" android:supportsRtl="true" android:theme="@style/AppTheme" > <activity android:name=".MainPage" android:label="@string/app_name" android:theme="@style/AppTheme.NoActionBar" > <intent-filter> <action android:name="android.intent.action.MAIN" /> <category android:name="android.intent.category.LAUNCHER" /> </intent-filter> </activity> <activity android:name=".UserList"> </activity> <activity android:name=".Post.PostData"> </activity> </application> </manifest>
Final output :
[maxbutton id=”1″]
I am getting
TypeError: Cannot read property “parameters” from undefined. (line 13, file “Code”) following error please help
Kindly share the screen shot of the code, I will try to solve by analysing it..
function doGet(e) {
return handleRequest(e);
}
function doPost(e){
return handleRequest(e);
}
function handleRequest(request){
var output = ContentService.createTextOutput();
var name = request.parameters.name;
var Sheet_ID = request.parameters.id;
// var name = request.parameter.Name;
// var email_id=request.parameter.Email_id;
// var Contact_No=request.parameter.Contact_No;
// var Place_of_Birth=request.parameter.Place_of_Birth;
// var DOB=request.parameter.DOB;
// var TOB=request.parameter.TOB;
// var Question=request.parameter.Question;
// var Payment=request.parameter.Payment;
// ,email_id,Contact_No,Place_of_Birth,DOB,TOB,Question,Payment
var spreadSheet = SpreadsheetApp.openById(Sheet_ID);
var sheet = spreadSheet.getSheetByName(“UserData”);
var rowCount = sheet.appendRow([name])
var callback = request.parameters.callback;
if (callback === undefined) {
output.setContent(JSON.stringify(“Success”));
} else {
output.setContent(callback + “(” + JSON.stringify(“Success”) + “)”);
}
output.setMimeType(ContentService.MimeType.JSON);
return output;
}
Hello Madur, Don’t run the function or project while u r using doGet or doPost.. Instead follow these steps
1) Go to Publish option
2) Deploy as web app
3) after deployment copy the url of deployed app
4) use post man tool given below to check by passing parameters. https://chrome.google.com/webstore/detail/postman/fhbjgbiflinjbdggehcddcbncdddomop?hl=en
for better understanding watch this video carefully.
https://www.youtube.com/watch?v=QZZrMlUiH2M
I follow the instructions as given in video but was getting the same error if you want to check you can contact to me on jmadhur.kothadar42@gmail.com
something wrong there man.
when I run the script tells me something like
TypeError: No se puede leer la propiedad “parameter” de undefined. (línea 20, archivo “Código”)
Hello Henris,
Don’t run the function or project while u r using doGet or doPost.. Instead follow these steps
1) Go to Publish option
2) Deploy as web app
3) after deployment copy the url of deployed app
4) use post man tool given below to check by passing parameters. https://chrome.google.com/w…
for better understanding watch this video carefully.
https://www.youtube.com/watch?v=QZZrMlUiH2M
hi. thanks for this. part 1 works for me. but when i add this add functionality. the app crash when i click the view user button and when im trying to add users the app give me false : 401 error. please help. thanks. im noob. sorry.
hi. thanks for this. part 1 works for me. but when i add this add
functionality. the app crash when i click the view user button and when
im trying to add users the app give me false : 401 error. please help.
thanks. im noob. sorry.
Download source code and try.. Just Change the spreadsheet ID in the step 2..i.e PostData.java class,
Try this with your package name and respective file name. It will work. Make changes in Android Manifest.
Great tutorial.
Thanks Madhur,
Can u make a tutorial for crud operation on spreadsheet from android app.
Sorry for late reply.. Will add it soon..
Nice tutorial.Really it will help us. It will be more helpful if you provide another tutorial where users can edit/delete his/her posted data.please help
Thanks Sushanta, Definitely I will help you in this regard. Will post it as soon as possible.
I am still waiting for your response.
sorry Sushant , will update by next week..
Ok..I will be waiting..
Hello Sushanta, sorry fr the delay you can refer this, will upload source code soon..http://www.androidlabs.info/uncategorized/google-sheet-as-database-for-your-android-application-part-3-crud-create-read-update-delete-operation-on-google-sheets-from-android-app/
Thank you.Really it is a great tutorial.
Wow you are really a genius. I tried several times to write CRUD code but failed everytime. Thank you with my whole heart for your kind support.
Sir, Can you please help in displaying the list using a scrollbar…..
Hello Nithin, the list view is having scroll bar in my example, So add more values and check, If you need any other help let me know.
Hello, I tried working… It is not possible to get expected results. Also when I am increasing number of values passed from App to Google spreadsheet, only value of the first parameter is taken and other three values are taken as undefined.
The output display activity which fetches more than 2 values from spreadsheet are working correctly. I observed that the data when inserted into spreadsheet, at that time only, value is taken as undefined or null. So please help me in fixxing….
Kindly share your code with google spread sheet screen shot or share the sheet.. to admin@androidlabs.info or you can post here only.
My app runs properly on my android device,but on submitting the records(name,country) it displays a message false : 401. What should i do ?……I tried it again by giving a proper spreadsheet id but it still shows the same error !!!!!!
Hello Soumil, Can you please send me the code. I will verify it. You can send me to admin@androidlabs.info or you can comment here only. So that others can refer it.
Sir, here is my main activity code and i’m getting the same error false 401.can you please clear the error.
Thanks in advance.
package com.example.personal.testingapp;
import android.app.ProgressDialog;
import android.os.AsyncTask;
import android.support.v7.app.AppCompatActivity;
import android.os.Bundle;
import android.util.Log;
import android.view.View;
import android.widget.Button;
import android.widget.EditText;
import android.widget.Toast;
import org.json.JSONObject;
import java.io.*;
import java.io.OutputStream;
import java.net.HttpURLConnection;
import java.net.URL;
import java.net.URLConnection;
import java.net.URLEncoder;
import java.util.Iterator;
import javax.net.ssl.HttpsURLConnection;
import static android.R.attr.button;
public class MainActivity extends AppCompatActivity {
private ProgressDialog progress;
EditText user_name;
EditText user_password;
Button button;
String name;
String password;
@Override
protected void onCreate(Bundle savedInstanceState)
{
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
button=(Button)findViewById(R.id.submit);
user_name=(EditText) findViewById(R.id.name);
user_password=(EditText) findViewById(R.id.password);
name = user_name.getText().toString();
password=user_password.getText().toString();
button.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
new SendRequest().execute();
}
} );
}
public class SendRequest extends AsyncTask {
protected void onPreExecute(){}
protected String doInBackground(String… arg0) {
try{
URL url = new URL(“https://script.google.com/macros/s/AKfycbyF6oMBFg2PSTdm8V0OUlUNQ8D8cQ0n0DYE_TnPjto8D108Q0o/exec”);
// https://script.google.com/macros/s/AKfycbyuAu6jWNYMiWt9X5yp63-hypxQPlg5JS8NimN6GEGmdKZcIFh0/exec
JSONObject postDataParams = new JSONObject();
//int i;
//for(i=1;i<=70;i++)
// String usn = Integer.toString(i);
String id= "1PoXTRrCUHCUY6IfOYY0KzitjI62ydwv7MrhnIfQgMxg";
postDataParams.put("name",name);
postDataParams.put("password",password);
postDataParams.put("id",id);
Log.e("params",postDataParams.toString());
HttpURLConnection conn = (HttpURLConnection) url.openConnection();
conn.setReadTimeout(15000 /* milliseconds */);
conn.setConnectTimeout(15000 /* milliseconds */);
conn.setRequestMethod("POST");
conn.setDoInput(true);
conn.setDoOutput(true);
OutputStream os = conn.getOutputStream();
BufferedWriter writer = new BufferedWriter(
new OutputStreamWriter(os, "UTF-8"));
writer.write(getPostDataString(postDataParams));
writer.flush();
writer.close();
os.close();
int responseCode=conn.getResponseCode();
if (responseCode == HttpsURLConnection.HTTP_OK)
{
BufferedReader in=new BufferedReader(new InputStreamReader(conn.getInputStream()));
StringBuffer sb = new StringBuffer("");
String line="";
while((line = in.readLine()) != null) {
sb.append(line);
break;
}
in.close();
return sb.toString();
}
else
{
return new String("false : "+responseCode);
}
}
catch(Exception e){
return new String("Exception: " + e.getMessage());
}
}
@Override
protected void onPostExecute(String result) {
Toast.makeText(getApplicationContext(), result,
Toast.LENGTH_LONG).show();
}
}
public String getPostDataString(JSONObject params) throws Exception {
StringBuilder result = new StringBuilder();
boolean first = true;
Iterator itr = params.keys();
while(itr.hasNext()){
String key= itr.next();
Object value = params.get(key);
if (first)
first = false;
else
result.append(“&”);
result.append(URLEncoder.encode(key, “UTF-8”));
result.append(“=”);
result.append(URLEncoder.encode(value.toString(), “UTF-8”));
}
return result.toString();
}
}
Its problem with app script. Can u share app script.
ReferenceError: “parameters” is not defined. (line 10, file “Code”, project “Test3”)
i am always getting this error plz help.
please pass the parameters.. use post man tool to do so Here is the link to install in chreome https://chrome.google.com/webstore/detail/postman/fhbjgbiflinjbdggehcddcbncdddomop?hl=en
1) After that insert your script web app url and pass the parameter..
2) Select Get or Post option then pass the parameters as defined in your script
https://uploads.disquscdn.com/images/a487368d76abe0a2c90a110b0e36026dfc053b484f957f080d579e1122dfa285.png
Refer this video.. for detailed information
https://www.youtube.com/edit?o=U&video_id=QZZrMlUiH2M
Hi! Any idea why my parameters that get added to my Spreadsheet appears as undefined? it does add the new row in the correct place but it appear as undefined for both columns.
It happens
1) if data type mismatch happens or
2) your Parameter name should match at both the side i.e in java code as well as in Script..
if possible share that java class from where you are sending data, i will try to solve. Also share your Script.
Hi! Yeah, I had an error with the names, was using uppercase instead of everything in lowercase and that messed it up. Thanks!! 😀 Awesome tutorial!
Thanks fr the response..
@@disqus_r1uSGCnq9d:disqus I am Also getting same error. how you rectified it
Hi there,
Thank you for sharing wonderful videos.
I believe you are a nice guy and intelligent too. I was wondering is it possible for you to assist me achieving something for me in your spare time absolutely similar to what you have done here.
What I am looking for is : information to flow from android to GSheet & reply the query too if possible. The data inserting , could it be entered using a scanner instead of doing it manually.
I will appreciate if you can reply to this request.
Many Thanks.
Pankaj
Ya sure.. Just mail your problem statement to admin@androidlabs.info
How to Insert the value of Radio button ?
Get the value from radio button, then pass it as parameter in postDataParams.put(“Parameter”,”value”) in PostData.java class.
Refer step 2 of Android Part in the above tutorial.
Hi, you tutorials are awesome. I am getting undefined error. can u please assist me where i went wrong?
My script
function doGet(e){
handleResponse(e);
}
//Recieve parameter and pass it to function to handle
function doPost(e){
handleResponse(e);
}
// here handle with parameter
function handleResponse(request) {
var output = ContentService.createTextOutput();
//create varibles to recieve respective parameters
var employeeid = request.parameter.employeeid;
var employeename=request.parameter.employeename;
var id = request.parameter.id;
var deviceos=request.parameter.deviceos;
var devicename=request.paramter.devicename;
var deviceid=request.parameter.deviceid;
var date=request.parameter.date;
var isreturn=request.paramater.isreturn;
//open your Spread sheet by passing id
var ss= SpreadsheetApp.openById(id);
var sheet=ss.getSheetByName(“Sheet1”);
//add new row with recieved parameter from client
var rowData = sheet.appendRow([employeeid,employeename,deviceos,devicename,deviceid,date,isreturn]);
var callback = request.parameters.callback;
if (callback === undefined) {
output.setContent(JSON.stringify(“Success”));
} else {
output.setContent(callback + “(” + JSON.stringify(“Success”) + “)”);
}
output.setMimeType(ContentService.MimeType.JSON);
return output;
}
My java code:
public class SendRequest extends AsyncTask {
protected void onPreExecute(){}
protected String doInBackground(String… arg0) {
try{
URL url = new URL(“myscripturl”);
JSONObject postDataParams = new JSONObject();
//int i;
//for(i=1;i<=70;i++)
// String usn = Integer.toString(i);
String id= "1xtnC61xYIRxrT_9ldYqqmukFLUpb4I0EDeWk6T-qWtU";
postDataParams.put("employeeid",employeeid);
postDataParams.put("employeename",employeename);
postDataParams.put("id",id);
postDataParams.put("deviceos",deviceos);
postDataParams.put("devicename",devicename);
postDataParams.put("deviceid",deviceid);
postDataParams.put("date",date);
postDataParams.put("isreturn","no");
Log.e("params",postDataParams.toString());
HttpURLConnection conn = (HttpURLConnection) url.openConnection();
conn.setReadTimeout(15000 /* milliseconds */);
conn.setConnectTimeout(15000 /* milliseconds */);
conn.setRequestMethod("POST");
conn.setDoInput(true);
conn.setDoOutput(true);
OutputStream os = conn.getOutputStream();
BufferedWriter writer = new BufferedWriter(
new OutputStreamWriter(os, "UTF-8"));
writer.write(getPostDataString(postDataParams));
writer.flush();
writer.close();
os.close();
int responseCode=conn.getResponseCode();
if (responseCode == HttpsURLConnection.HTTP_OK) {
BufferedReader in=new BufferedReader(new InputStreamReader(conn.getInputStream()));
StringBuffer sb = new StringBuffer("");
String line="";
while((line = in.readLine()) != null) {
sb.append(line);
break;
}
in.close();
return sb.toString();
}
else {
return new String("false : "+responseCode);
}
}
catch(Exception e){
return new String("Exception: " + e.getMessage());
}
}
@Override
protected void onPostExecute(String result) {
Toast.makeText(getApplicationContext(), result,
Toast.LENGTH_LONG).show();
}
}
public String getPostDataString(JSONObject params) throws Exception {
StringBuilder result = new StringBuilder();
boolean first = true;
Iterator itr = params.keys();
while(itr.hasNext()){
String key= itr.next();
Object value = params.get(key);
if (first)
first = false;
else
result.append(“&”);
result.append(URLEncoder.encode(key, “UTF-8”));
result.append(“=”);
result.append(URLEncoder.encode(value.toString(), “UTF-8”));
}
return result.toString();
}
}
i have facing error in multiple time in google postman please help me !!!!!!!!!!!!!!!
Bad value (line 17, file “Code”, project “test”)
Hello Dhananjay, sorry for late reply Can you send me the code to admin@androidlabs.info , I will check it and reply back
HI
How can i get data from google sheet to android app in form of listview. Please help me out
Refer other post in the blog , you will get.. Refer part 1
HI Android. I have get the data from google sheet inform of listview instead of editText. I have mailed you my Exact requirement to your mail. Please help me out.
My app runs properly on my android device,but on submitting the records(name,country) it displays a message false : 401. What should i do ?
Please send me the reply asap.
Check with internet connection, check in manifest , and check with the script by running it on browser ,
I’ve checked everything , It’s still showing the same . GET request is working but for POST , It’s giving 401.
Please Help
I applied the steps but I got this message when I wanted to Deploy as web app:
This app isn’t verified
This app hasn’t been verified by Google yet. Only proceed if you know and trust the developer.
Hi, It is just a formality. Google wants to verify you. See you are running your own code dont worry just proceed with it. Refer https://youtu.be/WuH-GBynC8U?t=229 for further steps
You can trust in your own.
what i have to do if i want to add check boxes
and add a row in the sheet that records automatically the date and time
plzz give me the code and steps to insert code
Hello
The script shown in the video is inserting only in two columns per row
No content is going in the third column
Please help me
You have to edit your script and catch the appropriate parameters.
Then you have to re-publish and select version as new.
Yes Carlos is right, if you are facing issues just mail us to
help@crazycodersclub.com
Hi,
I’d like to run the scripts across accounts. As an example, the script is published in xyz@gmail.com (developer) and when another user usr1@gmail.com log in app, I’d like the script runs over the usr1@gmail.com and not in xyz@gmail.com (that is what happening).
So do you have any suggestion how can I accomplish that?
when i am trying to insert value from app it shows “false 401”.I followed each and every step .please help me.
hello, first thanks for the information, I want to ask you a question, how would you use this code, if you have a system that you must obtain the String of the radioButton that the user chooses, then move on to another question, and must choose another RadioButton and so on , and at the end with a button you send all the data, Please Help me!
when i am trying to insert value from app it shows “false 401”.I followed each and every step .please help me.
source code download please
Works Perfect !!!